mirror of
https://github.com/soimort/you-get.git
synced 2025-02-11 12:42:29 +03:00
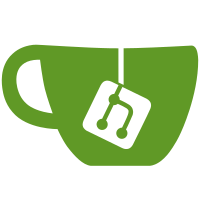
1. Introduce string.safe_chars, safe_print as ways to ensure that a string is encodable using the specified encoding. Unsafe characters are replaced with '?'. safe_print delegates to print and satisfies the same interface, so it can be used as a drop-in override for print in any file. 2. Move get_filename to fs, since that's where it belongs (fs-related filename handling). Move appending of ID, part number, and extension (when applicable) to get_filename, to avoid accidental truncation. 3. Remove common.tr, since the print override supercedes it. 4. Refactor of log module to work with changes (use print with different files instead of direct writes to stdout, stderr). 5. Modify other files to accommodate the changes (remove calls to tr) 6. Random cleanup I found: a. Some changes to impl of download_urls, download_urls_chunked (is this one even used?)). b. sina_download_by_id? c. ffmpeg_convert_ts_to_mkv tries to convert multiple input files onto the same output file, overwriting its own output each time? d. @staticmethod annotations (IDE sads otherwise). 7. Tests for the new encoding handling.
28 lines
1.4 KiB
Python
28 lines
1.4 KiB
Python
#!/usr/bin/env python
|
|
|
|
import unittest
|
|
|
|
from you_get.util.fs import *
|
|
|
|
class TestFs(unittest.TestCase):
|
|
def test_legitimize(self):
|
|
self.assertEqual(legitimize("1*2", os="Linux"), "1*2")
|
|
self.assertEqual(legitimize("1*2", os="Darwin"), "1*2")
|
|
self.assertEqual(legitimize("1*2", os="Windows"), "1-2")
|
|
|
|
def test_get_filename_simple(self):
|
|
self.assertEqual('name.ext', get_filename('name', 'ext', os='Linux', encoding='utf-8'))
|
|
|
|
def test_get_filename_parts(self):
|
|
self.assertEqual('name[02].ext', get_filename('name', 'ext', part=2, os='Linux', encoding='utf-8'))
|
|
self.assertEqual('name(02).ext', get_filename('name', 'ext', part=2, os='Windows', encoding='utf-8'))
|
|
|
|
def test_get_filename_encoding_error(self):
|
|
self.assertEqual('name\u20AC.ext', get_filename('name\u20AC', 'ext', os='Linux', encoding='utf-8'))
|
|
self.assertEqual('name\u20AC.ext', get_filename('name\u20AC', 'ext', os='Windows', encoding='utf-8'))
|
|
self.assertEqual('name?.ext', get_filename('name\u20AC', 'ext', os='Linux', encoding='ascii'))
|
|
self.assertEqual('name-.ext', get_filename('name\u20AC', 'ext', os='Windows', encoding='ascii'))
|
|
|
|
def test_get_filename_id(self):
|
|
self.assertEqual('name\u20AC.ext', get_filename('name\u20AC', 'ext', os='Linux', id='hi', encoding='utf-8'))
|
|
self.assertEqual('name? - hi.ext', get_filename('name\u20AC', 'ext', os='Linux', id='hi', encoding='ascii')) |